DynamicObject
DynamicObject
DynamicObject Reference
#include "TrueAxis.bi"
Inheritance diagram for DynamicObject:
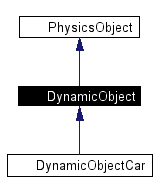
Description
Implements interactive objects in the physics simulation.
Call the function DynamicObjectCreateNew to create a new instance of this class.
All objects that need to react to physical forces should use this class in the simulation. DynamicObject inherits from PhysicsObject.
Initialising with CollisionObjectCombo allows for more complex collision objects. It also allows multiple dynamic objects to share the same collision objects to help reduce memory usage. The other initialisers are provided mostly for rapid prototyping purposes.
After the object has been initialised, various functions such as DynamicObjectSetMass can be used to set the objects properties.
Dynamics objects can be added and removed from the physics simulation by use of the functions PhysicsAddDynamicObject and PhysicsRemoveDynamicObject. The function DynamicObjectAddedToPhysics can be used to determine the current state.
- type DynamicObject extends PhysicsObject
- ...
- end type
- Functions:
- declare function DynamicObjectCreateNew() as DynamicObject ptr
- declare sub DynamicObjectRelease(byref pObj as const DynamicObject ptr )
- declare sub DynamicObjectInitialise(byval pObj as const DynamicObject ptr, byval pComboObj as const CollisionObjectCombo ptr)
- declare sub DynamicObjectInitialiseAsACube(byval pObj as const DynamicObject ptr, _
- byval centerX as single, byval centerY as single, byval centerZ as single, _
- byval size as single)
- declare sub DynamicObjectV3InitialiseAsACube(byval pObj as const DynamicObject ptr, byref v3Center as const TA_Vec3, byval size as single)
- declare sub DynamicObjectInitialiseAsAnOrientedCube(byval pObj as const DynamicObject ptr, _
- byval cx as single, byval cy as single, byval cz as single, _ ' center
- byval size as single, _
- byval px as single, byval py as single, byval pz as single, _ ' position
- byval pitch as single, byval yaw as single, byval roll as single)
- declare sub DynamicObjectV3InitialiseAsAnOrientedCube(byval pObj as const DynamicObject ptr, _
- byref v3Center as const TA_Vec3, _
- byval size as single, _
- byref v3Position as const TA_Vec3, _
- byval pitch as single, byval yaw as single, byval roll as single)
- declare sub DynamicObjectInitialiseAsABox(byval pObj as const DynamicObject ptr, _
- byval centerX as single, byval centerY as single, byval centerZ as single, _
- byval sizeX as single, byval sizeY as single, byval sizeZ as single)
- declare sub DynamicObjectV3InitialiseAsABox(byval pObj as const DynamicObject ptr, _
- byref v3Center as const TA_Vec3, _
- byref v3Extend as const TA_Vec3)
- declare sub DynamicObjectAABBInitialiseAsABox(byval pObj as const DynamicObject ptr, byref aabb as const TA_AABB)
- declare sub DynamicObjectInitialiseAsAnOrientedBox(byval pObj as const DynamicObject ptr, _
- byval cx as single, byval cy as single, byval cz as single, _ ' center
- byval w as single, byval h as single, byval d as single, _ ' size
- byval px as single, byval py as single, byval pz as single, _ ' position
- byval pitch as single, byval yaw as single, byval roll as single)
- declare sub DynamicObjectV3InitialiseAsAnOrientedBox(byval pObj as const DynamicObject ptr, _
- byref v3Center as const TA_Vec3, _ ' (most 0,0,0)
- byref v3Size as const TA_Vec3, _
- byref v3Position as const TA_Vec3, _
- byval pitch as single, byval yaw as single, byval roll as single)
- declare sub DynamicObjectAABBInitialiseAsAnOrientedBox(byval pObj as const DynamicObject ptr, _
- byref aabb as const TA_AABB, _ ' center and extend
- byref frame as const TA_MFrame) ' rotation and position
- declare sub DynamicObjectInitialiseAsASphere(byval pObj as const DynamicObject ptr, _
- byval centerX as single, byval centerY as single, byval centerZ as single, _
- byval radius as single)
- declare sub DynamicObjectV3InitialiseAsASphere(byval pObj as const DynamicObject ptr, byref v3Center as const TA_Vec3, byval radius as single)
- declare sub DynamicObjectInitialiseAsACylinder(byval pObj as const DynamicObject ptr, _
- byval startX as single, byval startY as single, byval startZ as single, _
- byval endX as single, byval endY as single, byval endZ as single, _
- byval radius as single)
- declare sub DynamicObjectV3InitialiseAsACylinder(byval pObj as const DynamicObject ptr, _
- byref v3PointA as const TA_Vec3, byref v3PointB as const TA_Vec3, byval radius as single)
- declare sub DynamicObjectInitialiseAsACapsule (byval pObj as const DynamicObject ptr, _
- byval startX as single, byval startY as single, byval startZ as single, _
- byval endX as single, byval endY as single, byval endZ as single, _
- byval radius as single)
- declare sub DynamicObjectV3InitialiseAsACapsule(byval pObj as const DynamicObject ptr, _
- byref v3PointA as const TA_Vec3, byref v3PointB as const TA_Vec3, byval radius as single)
- declare sub DynamicObjectSetUserData(byval pObj as const DynamicObject ptr, byval pUserData as const any ptr)
- declare function DynamicObjectGetUserData(byval pObj as const DynamicObject ptr) as any ptr
- ' helper only for DEBUG rendering by default all objects are visible
- declare sub DynamicObjectSetVisible(byval pObj as const DynamicObject ptr, byval bVisible as integer)
- declare function DynamicObjectIsVisible(byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectSetMass(byval pObj as const DynamicObject ptr, byval mass as single)
- declare function DynamicObjectGetMass(byval pObj as const DynamicObject ptr) as single
- declare function DynamicObjectGetInverseMass(byval pObj as const DynamicObject ptr) as single
- declare sub DynamicObjectSetCenterOffset(byval pObj as const DynamicObject ptr, byval x as single, byval y as single, byval z as single)
- declare sub DynamicObjectGetCenterOffset(byval pObj as const DynamicObject ptr, byref x as single, byref y as single, byref z as single)
- declare sub DynamicObjectV3SetCenterOffset(byval pObj as const DynamicObject ptr, byref v3CenterOffset as const TA_Vec3)
- declare sub DynamicObjectV3GetCenterOffset(byval pObj as const DynamicObject ptr, byref v3CenterOffset as TA_Vec3)
- declare sub DynamicObjectSetPosition(byval pObj as const DynamicObject ptr, byval x as single, byval y as single, byval z as single)
- declare sub DynamicObjectGetPosition(byval pObj as const DynamicObject ptr, byref x as single, byref y as single, byref z as single)
- declare sub DynamicObjectV3SetPosition(byval pObj as const DynamicObject ptr, byref v3Postion as const TA_Vec3)
- declare sub DynamicObjectV3GetPosition(byval pObj as const DynamicObject ptr, byref v3Postion as TA_Vec3)
- declare sub DynamicObjectSetRotation(byval pObj as const DynamicObject ptr, byval pitchRad as single, byval yawRad as single, byval rollRad as single)
- declare sub DynamicObjectGetRotation(byval pObj as const DynamicObject ptr, byref pitchRad as single, byref yawRad as single, byref rollRad as single)
- declare sub DynamicObjectSetRotationD(byval pObj as const DynamicObject ptr, byval pitchDeg as single, byval yawDeg as single, byval rollDeg as single)
- declare sub DynamicObjectGetRotationD(byval pObj as const DynamicObject ptr, byref pitchDeg as single, byref yawDeg as single, byref rollDeg as single)
- declare sub DynamicObjectSetFriction(byval pObj as const DynamicObject ptr, byval fFriction as single)
- declare function DynamicObjectGetFriction(byval pObj as const DynamicObject ptr) as single
- ' -1 to +1
- declare sub DynamicObjectSetRestitution(byval pObj as const DynamicObject ptr, byval fRestitution as single)
- declare function DynamicObjectGetRestitution(byval pObj as const DynamicObject ptr) as single
- declare sub DynamicObjectSetGravityMult(byval pObj as const DynamicObject ptr, byval fGravityMult as single)
- declare function DynamicObjectGetGravityMult(byval pObj as const DynamicObject ptr) as single
- declare sub DynamicObjectSetInfiniteMass(byval pObj as const DynamicObject ptr, byval bInfiniteMass as integer)
- declare function DynamicObjectIsInfiniteMass (byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectSetRotationDisabled(byval pObj as const DynamicObject ptr, byval bRotationDisabled as integer)
- declare function DynamicObjectIsRotationDisabled (byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectSetMovementDisabled(byval pObj as const DynamicObject ptr, byval bMovementDisabled as integer)
- declare function DynamicObjectIsMovementDisabled (byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectSetForceFastSolverEnabled(byval pObj as const DynamicObject ptr, byval bFastSolverEnabled as integer)
- declare function DynamicObjectIsForceFastSolverEnabled (byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectSetAllDampingDisabled(byval pObj as const DynamicObject ptr, byval bAllDampingDisabled as integer)
- declare function DynamicObjectIsAllDampingDisabled (byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectSetExtraStability(byval pObj as const DynamicObject ptr,byval bExtraStability as integer)
- declare function DynamicObjectIsExtraStability (byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectSetRigidFrictionDisabled(byval pObj as const DynamicObject ptr, byval bRigidFrictionDisabled as integer)
- declare function DynamicObjectIsRigidFrictionDisabled (byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectSetCollisionDisabled(byval pObj as const DynamicObject ptr, byval bCollisionDisabled as integer)
- declare function DynamicObjectIsCollisionDisabled (byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectSetUpdateDisabled(byval pObj as const DynamicObject ptr, byval bUpdateDisabled as integer)
- declare function DynamicObjectIsUpdateDisabled (byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectSetGhost(byval pObj as const DynamicObject ptr, byval bGhost as integer)
- declare function DynamicObjectIsGhost (byval pObj as const DynamicObject ptr) as integer
- declare function DynamicObjectIsWorldObject(byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectSetRestTimeMultiplier(byval pObj as const DynamicObject ptr, byval fRestTimeMultiplier as single)
- declare function DynamicObjectGetRestTimeMultiplier(byval pObj as const DynamicObject ptr) as single
- declare function DynamicObjectIsInMovingList(byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectSetToMoving (byval pObj as const DynamicObject ptr, byval priority as ePHYSICS_MOVING_PRIORITIES)
- declare sub DynamicObjectSetToResting(byval pObj as const DynamicObject ptr)
- declare sub DynamicObjectSetLinearVelocity(byval pObj as const DynamicObject ptr, byval x as single, byval y as single, byval z as single)
- declare sub DynamicObjectGetLinearVelocity(byval pObj as const DynamicObject ptr, byref x as single, byref y as single, byref z as single)
- declare sub DynamicObjectV3SetLinearVelocity(byval pObj as const DynamicObject ptr, byref v3LinearVelocity as const TA_Vec3)
- declare sub DynamicObjectV3GetLinearVelocity(byval pObj as const DynamicObject ptr, byref v3LinearVelocity as TA_Vec3)
- declare sub DynamicObjectSetAngularVelocity(byval pObj as const DynamicObject ptr, byval x as single, byval y as single, byval z as single)
- declare sub DynamicObjectGetAngularVelocity(byval pObj as const DynamicObject ptr, byref x as single, byref y as single, byref z as single)
- declare sub DynamicObjectV3SetAngularVelocity(byval pObj as const DynamicObject ptr, byref v3AngularVelocity as const TA_Vec3)
- declare sub DynamicObjectV3GetAngularVelocity(byval pObj as const DynamicObject ptr, byref v3AngularVelocity as TA_Vec3)
- declare sub DynamicObjectGetVelocityAtWorldPosition(byval pObj as const DynamicObject ptr, _
- byval worldX as single, byval worldY as single, byval worldZ as single, _
- byref velX as single, byref velY as single, byref velZ as single)
- declare sub DynamicObjectV3GetVelocityAtWorldPosition(byval pObj as const DynamicObject ptr, byref v3WorldPos as const TA_Vec3, byref v3Velocity as TA_Vec3)
- declare sub DynamicObjectApplyImpulse(byval pObj as const DynamicObject ptr, _
- byval impX as single, byval impY as single, byval impZ as single, _
- byval worldX as single, byval worldY as single, byval worldZ as single)
- declare sub DynamicObjectV3ApplyImpulse(byval pObj as const DynamicObject ptr, byref v3Impulse as const TA_Vec3, byref v3WorldPos as const TA_Vec3)
- declare sub DynamicObjectApplyLinearImpulse(byval pObj as const DynamicObject ptr, _
- byval impX as single, byval impY as single, byval impZ as single)
- declare sub DynamicObjectV3ApplyLinearImpulse(byval pObj as const DynamicObject ptr, byref v3LinearImpulse as const TA_Vec3)
- declare sub DynamicObjectApplyAngularImpulse(byval pObj as const DynamicObject ptr, _
- byval impX as single, byval impY as single, byval impZ as single)
- declare sub DynamicObjectV3ApplyAngularImpulse(byval pObj as const DynamicObject ptr, byref v3AngularImpulse as const TA_Vec3)
- DynamicObject with CollisionObjectLineList
- declare function DynamicObjectGetNumLineCollisions(byval pObj as const DynamicObject ptr) as integer
- declare function DynamicObjectGetLineCollision(byval pObj as const DynamicObject ptr, byval nLineIndex as integer) as LineIntersection ptr
- declare function DynamicObjectLineCollisionsGetIntersectionDepth(byval pObj as const DynamicObject ptr, byval nLineIndex as integer) as single
- declare function DynamicObjectLineCollisionsGetAttribute(byval pObj as const DynamicObject ptr, byval nLineIndex as integer) as integer
- declare sub DynamicObjectLineCollisionsGetPosition(byval pObj as const DynamicObject ptr, byval nLineIndex as integer, byref x as single, byref y as single, byref z as single)
- declare sub DynamicObjectLineCollisionsV3GetPosition(byval pObj as const DynamicObject ptr, byval nLineIndex as integer, byref v3Position as TA_Vec3)
- ' v3WorldPosition = LineCollisionsGetPosition() * DynamicObjectGetFrame()
- declare sub DynamicObjectLineCollisionsGetWorldPosition(byval pObj as const DynamicObject ptr, byval nLineIndex as integer, byref x as single, byref y as single, byref z as single)
- declare sub DynamicObjectLineCollisionsV3GetWorldPosition(byval pObj as const DynamicObject ptr, byval nLineIndex as integer, byref v3Position as TA_Vec3)
- declare sub DynamicObjectLineCollisionsGetNormal(byval pObj as const DynamicObject ptr, byval nLineIndex as integer, byref x as single, byref y as single, byref z as single)
- declare sub DynamicObjectLineCollisionsV3GetNormal(byval pObj as const DynamicObject ptr, byval nLineIndex as integer, byref v3Normal as TA_Vec3)
- declare function DynamicObjectLineCollisionsGetDynamicObject(byval pObj as const DynamicObject ptr, byval nLineIndex as integer) as DynamicObject ptr
- declare function DynamicObjectLineCollisionsGetCollisionObjectType(byval pObj as const DynamicObject ptr, byval nLineIndex as integer) as eCOLLISIONOBJECT_TYPES
- declare sub DynamicObjectAdjustLineCollisionFromNextFrame(byval pObj as const DynamicObject ptr, byval nLineIndex as integer)
- DynamicObject Joint
- declare function DynamicObjectAddWorldJoint(byval pObj as const DynamicObject ptr) as PhysicsJoint ptr
- declare function DynamicObjectAddJoint(byval pObj1 as const DynamicObject ptr, byval pObj2 as const DynamicObject ptr) as PhysicsJoint ptr
- declare sub DynamicObjectRemoveJoint(byval pObj as const DynamicObject ptr, byval pJoint as const PhysicsJoint ptr)
- declare sub DynamicObjectRemoveAllJoints(byval pObj as const DynamicObject ptr)
- DynamicObject Usergroup
- declare function DynamicObjectCreateUserGroup(byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectSetUserGroup(byval pObj as const DynamicObject ptr, byval nUserGroup as integer)
- declare function DynamicObjectGetUserGroup(byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectSetUserGroupItemId(byval pObj as const DynamicObject ptr, byval nItemId as integer)
- declare function DynamicObjectGetUserGroupItemId(byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectDisallowCollisionWithUserGroupItemId(byval pObj as const DynamicObject ptr, byval nItemId as integer)
- declare sub DynamicObjectAllowCollisionWithUserGroupItemId(byval pObj as const DynamicObject ptr, byval nItemId as integer)
- declare function DynamicObjectIsAllowedToCollideWith(byval pObjA as const DynamicObject ptr, byval pObjB as const DynamicObject ptr) as integer
- DynamicObject physic simualtion
- ' optional for advanced users
- ' use it inside of the DynamicObjectTemplate UpdateCallback
- ' step 1. Make sure that force and torque have been cleared.
- declare sub DynamicObjectAssertForceAndTorqueCleared(byval pObj as const DynamicObject ptr)
- ' step 2. Do gravity
- declare sub DynamicObjectAccumulateGravity(byval pObj as const DynamicObject ptr)
- ' step 3. Apply a force to the dynamic object
- declare sub DynamicObjectAccumulateForceAndTorque(byval pObj as const DynamicObject ptr, _
- byval worldForceX as single, byval worldForceY as single, byval worldForceZ as single, _
- byval worldPosX as single, byval worldPosY as single, byval worldPosZ as single)
- declare sub DynamicObjectV3AccumulateForceAndTorque(byval pObj as const DynamicObject ptr, _
- byref v3WorldForce as const TA_Vec3, _
- byref v3WorldPosition as const TA_Vec3)
- ' step 4. Apply the forces to linear and angular velocity
- declare sub DynamicObjectApplyForceAndTorqueToVelocities(byval pObj as const DynamicObject ptr, byval fDt as single)
- ' step 5. Clear force and torque (kg.m/s2).
- declare sub DynamicObjectClearForceAndTorque(byval pObj as const DynamicObject ptr)
- ' step 6. Update date the next frame of the dynamic object, ready for collision testing.
- declare sub DynamicObjectApplyVelocityToNextFrame(byval pObj as const DynamicObject ptr, byval fDt as single)
- declare sub DynamicObjectApplyVelocityFromNextFrame(byval pObj as const DynamicObject ptr, byval fDt as single)
- ' step 7. optional if the DynamicObject used a CollisionObjectLineList
- declare sub DynamicObjectClearLineIntersections(byval pObj as const DynamicObject ptr)
- ' ApplyNextFrame will be called to by Update automatically after the collision response has been done.
- declare sub DynamicObjectApplyNextFrame(byval pObj as const DynamicObject ptr)
- declare sub DynamicObjectAccumulateLinearForce(byval pObj as const DynamicObject ptr, _
- byval worldForceX as single, byval worldForceY as single, byval worldForceZ as single)
- declare sub DynamicObjectV3AccumulateLinearForce(byval pObj as const DynamicObject ptr, byref v3WorldForce as const TA_Vec3)
- declare sub DynamicObjectSetFindVelocityFromNextFrame(byval pObj as const DynamicObject ptr, byval bEnable as integer)
- declare function DynamicObjectGetFindVelocityFromNextFrame(byval pObj as const DynamicObject ptr) as integer
- declare sub DynamicObjectUpdateWorldSpaceInertialTensor(byval pObj as const DynamicObject ptr)
- declare sub DynamicObjectGetWorldAABB(byval pObj as const DynamicObject ptr, byref aabb as TA_AABB)
- declare sub DynamicObjectSetFrame(byval pObj as const DynamicObject ptr, byref frame as const TA_MFrame)
- declare sub DynamicObjectSetFrameDirect(byval pObj as const DynamicObject ptr, byref frame as const TA_MFrame)
- declare sub DynamicObjectGetFrame(byval pObj as const DynamicObject ptr, byref frame as TA_MFrame)
- declare sub DynamicObjectGetNextFrame(byval pObj as const DynamicObject ptr, byref frame as TA_MFrame)
- declare sub DynamicObjectGetPreviousFrame(byval pObj as const DynamicObject ptr, byref frame as TA_MFrame)
- declare sub DynamicObjectGetGraphicsFrame(byval pObj as const DynamicObject ptr, byref frame as TA_MFrame)
- declare sub DynamicObjectGetCenterOfMass (byval pObj as const DynamicObject ptr, byref v3CenterOfMass as TA_Vec3)
- declare sub DynamicObjectGetNextCenterOfMass(byval pObj as const DynamicObject ptr, byref v3CenterOfMass as TA_Vec3)
- declare sub DynamicObjectGetFrameRotation (byval pObj as const DynamicObject ptr, byref m33 as TA_Mat33)
- declare sub DynamicObjectGetFrameRotationV3X(byval pObj as const DynamicObject ptr, byref v3X as TA_Vec3)
- declare sub DynamicObjectGetFrameRotationV3Y(byval pObj as const DynamicObject ptr, byref v3Y as TA_Vec3)
- declare sub DynamicObjectGetFrameRotationV3Z(byval pObj as const DynamicObject ptr, byref v3Z as TA_Vec3)
- declare sub DynamicObjectGetNextFrameRotation (byval pObj as const DynamicObject ptr, byref m33 as TA_Mat33)
- declare sub DynamicObjectGetNextFrameRotationV3X(byval pObj as const DynamicObject ptr, byref v3X as TA_Vec3)
- declare sub DynamicObjectGetNextFrameRotationV3Y(byval pObj as const DynamicObject ptr, byref v3Y as TA_Vec3)
- declare sub DynamicObjectGetNextFrameRotationV3Z(byval pObj as const DynamicObject ptr, byref v3Z as TA_Vec3)
- declare sub DynamicObjectGetFrameRotationTransposeAsInverse(byval pObj as const DynamicObject ptr, byref m33 as TA_Mat33)
- DynamicObject Tools
- declare sub DynamicObjectGetOpenGLMatrix(byval pObj as const DynamicObject ptr, byval pMatrix4x4 as const single ptr)
- declare sub DynamicObjectGetOpenGLFrame (byval pObj as const DynamicObject ptr, byref frame as TA_MFrame)
- #ifdef USEOPENGL
- declare sub DynamicObjectMultOpenGLMatrix(byval pObj as const DynamicObject ptr)
- #else
- #define DynamicObjectMultOpenGLMatrix(pObj) :
- #endif
- ' out=in*DynamicObjectGetFrame().Rotation()
- declare sub DynamicObjectRotate(byval pObj as const DynamicObject ptr, _
- byval inX as single, byval inY as single, byval inZ as single, _
- byref outX as single, byref outY as single, byref outZ as single)
- declare sub DynamicObjectV3Rotate(byval pObj as const DynamicObject ptr, _
- byref v3In as const TA_Vec3, _
- byref v3Out as TA_Vec3)
- ' out=in*DynamicObjectGetFrame().
- declare sub DynamicObjectTransform(byval pObj as const DynamicObject ptr, _
- byval inX as single, byval inY as single, byval inZ as single, _
- byref outX as single, byref outY as single, byref outZ as single)
- declare sub DynamicObjectV3Transform(byval pObj as const DynamicObject ptr, _
- byref v3Vector as const TA_Vec3, _
- byref v3Result as TA_Vec3)
Copyright © 2015
Created with the Freeware Edition of HelpNDoc: Easily create Help documents